Usando Um Arduino® Para Controlar Dispositivos Bluetooth
Um pequeno projecto de 10 minutos para poder controlar dispositivos Bluetooth.
Material Necessário:
- Software Arduino IDE (https://www.arduino.cc/en/software);
- Um diapositivo Android, iOS ou um PC com Bluetooth v4.0 ou superior;
- No caso do SO Android ou iOS, necessita instalar a app RoboRemoFree (https://www.roboremo.app) no dispositivo.
Hardware + Componentes Electrónicos:
- 1x Arduino UNO (ou clone 100% compatível);
- 1x módulo Bluetooth HM-10 (ou clone 100% compatível);
- 1x resistência de 1K ohms 1/4 de watt;
- 1x resistência de 2K ohms 1/4 de watt;
- 1x resistência de 220 ohms 1/4 de watt;
- 1x LED de 3 mm ou 5 mm (qualquer cor);
- 1x breadboard;
- Vários fios eléctricos para ligar os componentes.
Esquema/Diagrama de Ligação:
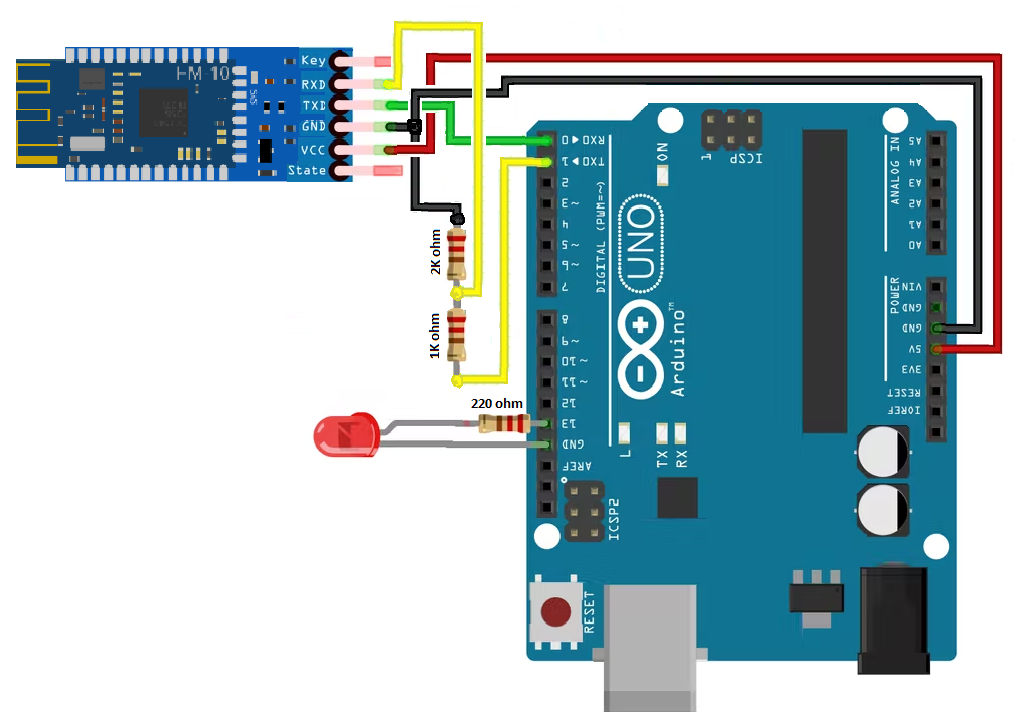
Código/Sketch:
----------------------- Bluetooth.ino -----------------------
///////////////////////////////////////////////////////////////////////////////
// Project: Bluetooth LED
// URL:
// File: Bluetooth.ino
//
// Description:
// This Sketch controls a LED connected to an Arduino via
// Bluetooth (supplied by an HM-10 Bluetooth module), from an app.
//
// Copyright (C) 2021-2022:
// José Caetano Silva / CaetanoSoft
//
// License:
// This file is part of Bluetooth LED.
//
// Bluetooth LED is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Bluetooth LED is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with Bluetooth LED. If not, see <https://www.gnu.org/licenses/>.
///////////////////////////////////////////////////////////////////////////////
// Remove comment from next line to use Software Serial
// instead of Hardware Serial
//#define SOFTSERIAL
#ifdef SOFTSERIAL
#include <SoftwareSerial.h>
// Bluetooth RX pin on Arduino
#define BT_RX_PIN = 10
// Bluetooth TX pin on Arduino
#define BT_TX_PIN = 11
SoftwareSerial SerialBT(BT_RX_PIN, BT_TX_PIN);
#else
HardwareSerial SerialBT = Serial1;
#endif
// The LED anode (+) pin on Arduino
#define LED_PIN 14
int ledPin = LED_PIN; // The LED anode (+) pin on Arduino
bool ledState = false; // State: false => Off; true => On
// Incomming Bluetooth message from the external controler device
String incomingMessage = "";
/**
* Change the LED on or off.
*/
void ChangeLED(int ledPin, bool ledState)
{
if (ledState == false)
{
// Turn LED OFF
digitalWrite(ledPin, LOW);
// Send back, to the Bluetooth device, the message "LED: OFF"
SerialBT.println("LED: OFF");
#ifndef SOFTSERIAL
// Send debug OK message to the console, the string "OK: LED (pin *) OFF"
Serial.print("OK: LED (pin ");
Serial.print(ledPin);
Serial.println(") OFF");
#endif
}
else
{
// Turn LED ON
digitalWrite(ledPin, HIGH);
// Send back, to the Bluetooth device, the message "LED: ON"
SerialBT.println("LED: ON");
#ifndef SOFTSERIALON
// Send debug OK message to the console, the string "OK: LED (pin *) ON"
Serial.print("OK: LED (pin ");
Serial.print(ledPin);
Serial.println(") ON");
#endif
}
}
/**
* Processes the Bluetooth command message recived
*/
bool ProcessBluetoothCommand(String incomingMessage)
{
bool retVal = false;
if(incomingMessage == "0")
{
ledPin = LED_PIN;
ledState = false;
retVal = true;
}
else if(incomingMessage == "1")
{
ledPin = LED_PIN;
ledState = true;
retVal = true;
}
else
{
retVal = false;
}
return retVal;
}
/**
* The setup function runs once when you press reset or power the board.
*/
void setup()
{
// Serial communications initialization
#ifdef SOFTSERIAL
SerialBT.begin(9600); // Default software communication baud rate of the Bluetooth module
Serial.begin(9600); // Default communication baud rate to the console
#else
SerialBT.begin(38400); // Default hardware communication baud rate of the Bluetooth module
Serial.begin(9600); // Default communication baud rate to the console
#endif
// LED initialization (off)
pinMode(LED_PIN, OUTPUT);
digitalWrite(LED_PIN, LOW);
}
/**
* The loop function runs over and over again forever.
*/
void loop()
{
// Checks whether data is comming from the BlueToorh Serial Port
if(SerialBT.available() > 0)
{
// Read the incoming data string message
incomingMessage = SerialBT.readString();
// Process the BlueToorh command recived
if(ProcessBluetoothCommand(incomingMessage))
{
ChangeLED(ledPin, ledState);
}
else
{
// Send back, to the Bluetooth device, the message "ERROR BAD COMMAND"
Serial.println("ERROR BAD COMMAND");
#ifndef SOFTSERIAL
// Send debug error message to the console
Serial.print("ERROR: Unknown command '");
Serial.print(incomingMessage);
Serial.println("' recived");
#endif
}
}
}
----------------------- Bluetooth.ino -----------------------
Instruções de Montagem:
Arduino Uno | Voltage Divider | Bluetooth HM-10 |
---|---|---|
RX (Pin 0) | <------> | TX |
TX (Pin 1) | <--> Center <--> | RX |
GND | <------> | GND |
5V | <------> | VCC |
Arduino Uno | LED |
---|---|
GND | Cathod (-) |
Pin 13 (Digital) | Anode (+) |
Faça as conexões entre os componentes como é mostrado nas tabelas 1 e 2 acima e no diagrama de ligação. Tenha a atenção que o módulo Bluetooth HM-10 têm uma voltagem de alimentação (VCC) entre os 3.3V e os 5V, mas funciona internamente a 3,3V (aceita entre os 2.0V e os 3,7V), ou seja os sinais RX e TX no módulo HM-10 são de 0V ou 3,3V mas no Arduino são de 0V ou 5V. Por isso é usado um divisor de tensão (ver esquema) para ligar os pinos Arduino TX <--> HM-10 RX (converte os 5V para 3,3V). A ligação dos pinos Arduino RX <--> HM-10 TX, não precisa de conversão, pois o Arduino aceita os 3,3V do HM-10 como um 1 binário.
Caso ainda não tenha instalado, instale no Arduino IDE, as seguintes bibliotecas, instalando todas as dependências:
* Wire;
* SoftwareSerial.
Use o Arduino IDE para colar o sketch "Bluetooth.ino" e gravar-lo, depois compile-o e envie-o para o dispositivo Arduino Uno.
Use a app RoboRemoFree para criar uma interface que envie uma string "1" para ligar o LED ou "0" para o desligar.
Funcionamento:
Ao alimentar o seu Arduino com tensão, este ficará à espera de comandos via Bluetooh de outro dispositivo para ligar/desligar o LED.